added firststeps + reorganized website a bit
This commit is contained in:
parent
4e7e5d24db
commit
3df44b76a4
22
_quarto.yml
22
_quarto.yml
@ -2,6 +2,8 @@ project:
|
||||
type: "website"
|
||||
title: "Research Software Engineering Summer School"
|
||||
|
||||
editor:
|
||||
render-on-save: true
|
||||
|
||||
website:
|
||||
title: "Research Software Engineering Summer School"
|
||||
@ -12,17 +14,27 @@ website:
|
||||
location: navbar
|
||||
type: textbox
|
||||
sidebar:
|
||||
collapse-level: 3
|
||||
style: docked
|
||||
contents:
|
||||
- href: index.qmd
|
||||
text: "🏠 Home"
|
||||
#- href: schedule.qmd
|
||||
# text: "📓 Schedule"
|
||||
- href: schedule.qmd
|
||||
text: "📓 Schedule"
|
||||
- href: teaching.qmd
|
||||
text: "📟 Teachers"
|
||||
- href: application.qmd
|
||||
text: "✍️ Apply Now"
|
||||
|
||||
- section: "Slides / Handouts"
|
||||
contents:
|
||||
- section: "Monday"
|
||||
contents:
|
||||
- href: material/1_mon/rse/rse_basics_slides.qmd
|
||||
text: "📊 1 - RSE"
|
||||
- href: "missing.md"
|
||||
text: "2 - Why Julia"
|
||||
- href: "material/1_mon/firststeps/firststeps_handout.qmd"
|
||||
text: "📝 3 - First Steps"
|
||||
- href: "material/1_mon/envs/envs_handout.qmd"
|
||||
text: "📝 4 - Environments & Packages"
|
||||
navbar:
|
||||
background: primary
|
||||
page-footer:
|
||||
|
@ -1,27 +0,0 @@
|
||||
---
|
||||
title: "Applications"
|
||||
---
|
||||
|
||||
::: callout-note
|
||||
Application (most likely) opens in mid-June 2023
|
||||
:::
|
||||
|
||||
### Requirements
|
||||
- one programming language on at least intermediate level [^1] (does **not** need to be Julia)
|
||||
- Basics of GIT (only! - see below)
|
||||
|
||||
### Important Dates
|
||||
- mid-June 2023 Application opens
|
||||
- mid-July 2023 Invites are send out
|
||||
- 31 July 2023 Time to confirm and pay (students on waiting-list are informed)
|
||||
|
||||
### Application Process
|
||||
There is a **two-stage** application process: first you apply - then we select (to slightly titrate a common starting-skill level) - then you get invited to confirm which includes the payment-process.
|
||||
|
||||
### Motivation
|
||||
We will ask for a short (500 chars) motivation statement.
|
||||
|
||||
For example you could state why this course will be helpful to your PhD project, why this is the right time for you to learn these skills to you or why you are excited for this topic. No need to answer all (or even any) of these questions.
|
||||
|
||||
|
||||
[^1]: While we know it is near impossible to gauge skills adequately, we still want to have some assurance on the minimal programming experience/skill level. We will ask to self-identify as **Beginner** (e.g. experience from courses, knows basic syntax), **Advanced Beginner** (did some analyses, basic plotting is no longer a problem), **Intermediate** (used it to complete a project/paper, can decode many of the error messages), **Advanced** (developed packages, can debug 90% of problems, helps others).
|
0
material/1_mon/envs/envs_handout.qmd
Normal file
0
material/1_mon/envs/envs_handout.qmd
Normal file
417
material/1_mon/firststeps/firststeps_handout.qmd
Normal file
417
material/1_mon/firststeps/firststeps_handout.qmd
Normal file
@ -0,0 +1,417 @@
|
||||
---
|
||||
code-annotations: select
|
||||
---
|
||||
# First Steps
|
||||
|
||||
## Getting started
|
||||
|
||||
::: callout-tip
|
||||
The [julia manual](https://docs.julialang.org/en/v1/manual/getting-started/) is excellent!
|
||||
:::
|
||||
|
||||
At this point we assume that you have Julia 1.9 installed, VSCode ready, and installed the VSCode Julia plugin. There are some more [recommended settings in VSCode](vscode.qmd) which are not necessary, but helpful.
|
||||
|
||||
We further recommend to not use the small "play" button on the top right (which opens a new julia process everytime you change something), but rather open a new Julia repl (`ctrl`+`shift`+`p` => `>Julia: Start Repl`) which you keep open as long as possible.
|
||||
|
||||
::: callout-tip
|
||||
VSCode automatically loads the `Revise.jl` package, which screens all your actively loaded packages/files and updates the methods instances whenever it detects a change. This is quite similar to `%autorelad 2` in python. If you use VSCode, you dont need to think about it, if you prefer a command line, you should put Revise.jl in your startup.jl file.
|
||||
:::
|
||||
|
||||
|
||||
## Syntax differences Python/R/MatLab
|
||||
|
||||
### In the beginning there was `nothing`
|
||||
`nothing`- but also `NaN` and also `Missing`.
|
||||
|
||||
Each of those has a specific purpose, but most likely we will only need `a = nothing` and `b = NaN`
|
||||
|
||||
### Control Structures
|
||||
|
||||
**Matlab User?** Syntax will be *very* familiar.
|
||||
|
||||
**R User?** Forget about all the `{}` brackets
|
||||
|
||||
**Python User?** We don't need no intendation, and we also have 1-index
|
||||
|
||||
``` julia
|
||||
myarray = zeros(6) # <1>
|
||||
for k = 1:length(myarray) # <2>
|
||||
if iseven(k)
|
||||
myarray[k] = sum(myarray[1:k]) # <3>
|
||||
elseif k == 5
|
||||
myarray = myarray .- 1 # <4>
|
||||
else
|
||||
myarray[k] = 5
|
||||
end # <5>
|
||||
end
|
||||
```
|
||||
|
||||
1. initialize a vector (check with `typeof(myArray)`)
|
||||
2. Control-Structure for-loop. 1-index!
|
||||
3. **MatLab**: Notice the `[` brackets to index Arrays!
|
||||
4. **Python/R**: `.` always means elementwise
|
||||
5. **Python/R**: `end` after each control sequence
|
||||
|
||||
### Functions
|
||||
```julia
|
||||
function myfunction(a,b=123;keyword1="defaultkeyword") #<1>
|
||||
if keyword1 == "defaultkeyword"
|
||||
c = a+b
|
||||
else
|
||||
c= a*b
|
||||
end
|
||||
return c
|
||||
end
|
||||
methods(myfunction) # <2>
|
||||
myfunction(0)
|
||||
myfunction(1;keyword1 = "notdefault")
|
||||
myfunction(0,5)
|
||||
myfunction(0,5;keyword1 = "notdefault")
|
||||
```
|
||||
1. everything before the `;` => positional, after => `kwargs`
|
||||
2. returns two functions, due to the `b=123` optional positional argument
|
||||
|
||||
```julia
|
||||
anonym = (x,y) -> x+y
|
||||
anonym(3,4)
|
||||
```
|
||||
|
||||
```julia
|
||||
myshortfunction(x) = x^2
|
||||
function mylongfunction(x)
|
||||
return x^2
|
||||
end
|
||||
```
|
||||
|
||||
#### elementwise-function / broadcasting
|
||||
Julia is very neat in regards of applying functions elementwise (also called broadcasting). (Matlab users know this already).
|
||||
|
||||
```julia
|
||||
a = [1,2,3,4]
|
||||
b = sqrt(a) # <1>
|
||||
c = sqrt.(a) # <2>
|
||||
```
|
||||
1. Error - there is no method defined for the `sqrt` of an `Vector`
|
||||
2. the small `.` applies the function to all elements of the container `a` - this works as "expected"
|
||||
|
||||
::: callout-important
|
||||
Broadcasting is very powerful, as julia can get a huge performance boost in chaining many operations, without requiring saving temporary arrays. For example:
|
||||
```julia
|
||||
a = [1,2,3,4,5]
|
||||
b = [6,7,8,9,10]
|
||||
|
||||
c = (a.^2 .+ sqrt.(a) .+ log.(a.*b))./5
|
||||
```
|
||||
|
||||
In many languages (matlab, python, R) you would need to do the following:
|
||||
```
|
||||
1. temp1 = a.*b
|
||||
2. temp2 = log.(temp1)
|
||||
3. temp3 = a.^2
|
||||
4. temp4 = sqrt.(a)
|
||||
5. temp5 = temp3 .+ temp4
|
||||
6. temp6 = temp5 + temp2
|
||||
7. output = temp6./5
|
||||
```
|
||||
Thus, we need to allocate ~7x the memory of the vector (not at the same time though)
|
||||
|
||||
In Julia, the elementwise code above rather translates to:
|
||||
|
||||
```julia
|
||||
c = similar(a) # <1>
|
||||
for k = 1:length(a)
|
||||
c[k] = (a[k]^2 + sqrt(a[k]) + log(a[k]*b[k]))./5
|
||||
end
|
||||
|
||||
```
|
||||
1. Function to initialize an `undef` array with the same size as `a`
|
||||
|
||||
The `temp` memory we need at each iteration is simply `c[k]`.
|
||||
And a nice sideeffect: by doing this, we get rid of any specialized "serialized" function e.g. to do sum, or + or whatever. Those are typically the inbuilt `C` functions in python/matlab/R, that really speed up things. In Julia **we do not need inbuilt functions for speed**.
|
||||
:::
|
||||
|
||||
|
||||
|
||||
## Style-conventions
|
||||
|
||||
| | |
|
||||
| -- | -- |
|
||||
| variables | lowercase, lower_case|
|
||||
| Types,Modules | UpperCamelCase|
|
||||
| functions, macro | lowercase |
|
||||
| inplace / side-effects | `endwith!()` |
|
||||
|
||||
# Task 1.
|
||||
Ok - lot of introduction, but I think you are ready for your first interactive task.
|
||||
|
||||
## Wait - how do I even run things in Julia/VScode?
|
||||
Typically, you work in a Julia script ending in `scriptname.jl`
|
||||
|
||||
You concurrently have a REPL open, to not reload all packages etc. everytime. Further you typically have `Revise.jl` running in the background to automatically update your custom Packages / Modules (more to that later).
|
||||
|
||||
You can mark some code and execute it using `ctrl` + `enter` - you can also generate code-blocks using `#---` and run a whole code-block using `alt`+`enter`
|
||||
|
||||
1. Open a new script `statistic_functions.jl` in VSCode in a folder of your choice.
|
||||
|
||||
2. implement a function called `rse_sum`^[rse = research software engineering, we could use `sum` in a principled way, but it requires some knowledge you likely don't have right now]. This function should return `true` if provided with the following test: `res_sum(1:36) == 666`. You should further make use of a for-loop.
|
||||
|
||||
3. implement a second function called `rse_mean`, which calculates the mean of the provided vector. Make sure to use the `rse_sum` function! Test it using `res_mean(-15:17) == 1`
|
||||
|
||||
4. Next implement a standard deviation function `rse_std`: $\sqrt{\frac{\sum(x-mean(x))}{n-1}}$, this time you should use elementwise/broadcasting operators. Test it with `rse_std(1:3) == 1`
|
||||
|
||||
5. Finally, we will implement `rse_tstat`, returning the t-value with `length(x)-1` DF, that the provided Array actually has a mean of 0. Test it with `rse_tstat(2:3) == 5`. Add the keyword argument `σ` that allows the user to optionally provide a pre-calculated standard deviation.
|
||||
|
||||
Well done! You now have all functions defined with which we will continue our journey.
|
||||
|
||||
# Julia Basics - II
|
||||
### Strings
|
||||
|
||||
```julia
|
||||
character = 'a'
|
||||
str = "abc"
|
||||
str[3] # <1>
|
||||
```
|
||||
1. returns `c`
|
||||
|
||||
##### characters
|
||||
```julia
|
||||
'a':'f' #<1>
|
||||
collect('a':'f') # <2>
|
||||
join('a':'f') # <3>
|
||||
```
|
||||
1. a `StepRange` between characters
|
||||
2. a `Array{Chars}`
|
||||
3. a `String`
|
||||
|
||||
##### concatenation
|
||||
|
||||
```julia
|
||||
a = "one"
|
||||
b = "two"
|
||||
ab = a * b # <1>
|
||||
|
||||
```
|
||||
1. Indeed, `*` and not `+` - as plus implies from algebra that `a+b == b+a` which obviously is not true for string concatenation. But `a*b !== b*a` - at least for matrices.
|
||||
|
||||
##### substrings
|
||||
```julia
|
||||
str = "long string"
|
||||
substr = SubString(str, 1, 4)
|
||||
whereis_str = findfirst("str",str)
|
||||
```
|
||||
|
||||
##### regexp
|
||||
```julia
|
||||
str = "any WORD written in CAPITAL?"
|
||||
occursin(r"[A-Z]+", str) # <1>
|
||||
m = match(r"[A-Z]+",str) # <2>
|
||||
```
|
||||
1. Returns `true`. Note the small `r` before the `r"regular expression"` - nifty!
|
||||
2. Returns a `::RegexMatch` - access via `m.match` & `m.offset` (index) - or `m.captures` / `m.offsets` if you defined capture-groups
|
||||
|
||||
##### Interpolation
|
||||
```julia
|
||||
a = 123
|
||||
str = "this is a: $a; this 2*a: $(2*a)"
|
||||
```
|
||||
|
||||
## Scopes
|
||||
All things (excepts modules) are in local scope (in scripts)
|
||||
|
||||
``` julia
|
||||
a = 0
|
||||
for k = 1:10
|
||||
a = 1
|
||||
end
|
||||
a #<1>
|
||||
```
|
||||
1. a = 0! - in a script; but a = 1 in the REPL!
|
||||
|
||||
Variables are in global scope in the REPL for debugging convenience
|
||||
|
||||
::: callout-tip
|
||||
Putting this code into a function automatically resolves this issue
|
||||
```julia
|
||||
function myfun()
|
||||
a = 0
|
||||
for k = 1:10
|
||||
a = 1
|
||||
end
|
||||
a #<1>
|
||||
return a
|
||||
end
|
||||
myfun() # <1>
|
||||
```
|
||||
1. returns 1 now in both REPL and include("myscript.jl")
|
||||
|
||||
:::
|
||||
|
||||
#### explicit global / local
|
||||
|
||||
``` julia
|
||||
a = 0
|
||||
global b
|
||||
b = 0
|
||||
for k = 1:10
|
||||
local a
|
||||
global b
|
||||
a = 1
|
||||
b = 1
|
||||
end
|
||||
a #<1>
|
||||
b #<2>
|
||||
```
|
||||
|
||||
1. a = 0
|
||||
2. b = 1
|
||||
|
||||
|
||||
#### Modifying containers works in any case
|
||||
```julia
|
||||
a = zeros(10)
|
||||
for k = 1:10
|
||||
|
||||
a[k] = k
|
||||
end
|
||||
a #<1>
|
||||
```
|
||||
1. This works "correctly" in the `REPL` as well as in a script, because we modify the content of `a`, not `a` itself
|
||||
|
||||
## Types
|
||||
Types play a super important role in Julia for several main reasons:
|
||||
|
||||
1) The allow for specialization e.g. `+(a::Int64,b::Float64)` might have a different (faster?) implementation compared to `+(a::Float64,b::Float64)`
|
||||
2) They allow for generalization using `abstract` types
|
||||
3) They act as containers, structuring your programs and tools
|
||||
|
||||
Everything in julia has a type! Check this out:
|
||||
```julia
|
||||
typeof(1)
|
||||
typeof(1.0)
|
||||
typeof(sum)
|
||||
typeof([1])
|
||||
typeof([(1,2),"5"])
|
||||
```
|
||||
----
|
||||
|
||||
We will discuss two types of types:
|
||||
|
||||
1) **`composite`** types
|
||||
2) `abstract` types.
|
||||
|
||||
::: {.callout-tip collapse="true"}
|
||||
## Click me for even more types!
|
||||
There is a third type, `primitive type` - but we will practically never use them
|
||||
Not much to say at this level, they are types like `Float64`. You could define your own one, e.g.
|
||||
```julia
|
||||
primitive type Float128 <: AbstractFloat 128 end
|
||||
```
|
||||
|
||||
And there are two more, `Singleton types` and `Parametric types` - which (at least the latter), you might use at some point. But not in this tutorial.
|
||||
|
||||
:::
|
||||
|
||||
|
||||
### composite types
|
||||
You can think of these types as containers for your variables, which allows you for specialization.
|
||||
```julia
|
||||
struct SimulationResults
|
||||
parameters::Vector
|
||||
results::Vector
|
||||
end
|
||||
|
||||
s = SimulationResults([1,2,3],[5,6,7,8,9,10,NaN])
|
||||
|
||||
function print(s::SimulationResults)
|
||||
println("The following simulation was run:")
|
||||
println("Parameters: ",s.parameters)
|
||||
println("And we got results!")
|
||||
println("Results: ",s.results)
|
||||
end
|
||||
|
||||
print(s)
|
||||
|
||||
function SimulationResults(parameters) # <1>
|
||||
results = run_simulation(parameters)
|
||||
return SimulationResults(parameters,results)
|
||||
end
|
||||
|
||||
function run_simulation(x)
|
||||
return cumsum(repeat(x,2))
|
||||
end
|
||||
|
||||
s = SimulationResults([1,2,3])
|
||||
print(s)
|
||||
|
||||
|
||||
```
|
||||
1. in case not all fields are directly defined, we can provide an outer constructor (there are also inner constructors, but we will not discuss them here)
|
||||
|
||||
|
||||
::: callout-warning
|
||||
once defined, a type-definition in the global scope of the REPL cannot be re-defined without restarting the julia REPL! This is annoying, there are some tricks arround it (e.g. defining the type in a module (see below), and then reloading the module)
|
||||
:::
|
||||
|
||||
# Task 2
|
||||
|
||||
1. Implement a type `StatResult` with fields for `x`, `n`, `std` and `tvalue`
|
||||
2. Implement an outer constructor that can run `StatResult(2:10)` and return the full type including the calculated t-values.
|
||||
3. Implement a function `length` for `StatResult` to multiple-dispatch on
|
||||
4. **Optional:** If you have time, optimize the functions, so that mean, sum, length, std etc. is not calculated multiple times - you might want to rewrite your type. Note: This is a bit tricky :)
|
||||
|
||||
# Julia Basics III
|
||||
## Modules
|
||||
```julia
|
||||
module MyStatsPackage
|
||||
include("src/statistic_functions.jl")
|
||||
export SimulationResults #<1>
|
||||
export rse_tstat
|
||||
end
|
||||
|
||||
using MyStatsPackage
|
||||
|
||||
```
|
||||
1. This makes the `SimulationResults` type immediately available after running `using MyStatsPackage`. To use the other "internal" functions, one would use `MyStatsPackage.rse_sum`.
|
||||
```julia
|
||||
import MyStatsPackage
|
||||
|
||||
MyStatsPackage.rse_tstat(1:10)
|
||||
|
||||
import MyStatsPackage: rse_sum
|
||||
rse_sum(1:10)
|
||||
```
|
||||
|
||||
## Macros
|
||||
Macros allow to programmers to edit the actual code **before** it is run. We will pretty much just use them, without learning how they work.
|
||||
|
||||
```julia
|
||||
@which cumsum
|
||||
@which(cumsum)
|
||||
a = "123"
|
||||
@show a
|
||||
```
|
||||
# Cheatsheets
|
||||
|
||||
## meta-tools
|
||||
|
||||
<!-- maybe move to own file "cheatsheets?" -->
|
||||
|
||||
| | Julia | Python |
|
||||
|------------------------|------------------------|------------------------|
|
||||
| Documentation | `?obj` | `help(obj)` |
|
||||
| Object content | `dump(obj)` | `print(repr(obj))` |
|
||||
| Exported functions | `names(FooModule)` | `dir(foo_module)` |
|
||||
| List function signatures with that name | `methods(myFun)` | |
|
||||
| List functions for specific type | `methodswith(SomeType)` | `dir(SomeType)` |
|
||||
| Where is ...? | `@which func` | `func.__module__` |
|
||||
| What is ...? | `typeof(obj)` | `type(obj)` |
|
||||
| Is it really a ...? | `isa(obj, SomeType)` | `isinstance(obj, SomeType)` |
|
||||
|
||||
## debugging
|
||||
|||
|
||||
|--|--|
|
||||
`@run sum(5+1)`| run debugger, stop at error/breakpoints
|
||||
`@enter sum(5+1)` | enter debugger, dont start code yet
|
||||
`@show variable` | prints: variable = variablecontent
|
||||
`@debug variable` | prints only to debugger, very convient in combination with `>ENV["JULIA_DEBUG"] = ToBeDebuggedModule` (could be `Main` as well)
|
||||
|
51
material/1_mon/firststeps/statistic_functions.jl
Normal file
51
material/1_mon/firststeps/statistic_functions.jl
Normal file
@ -0,0 +1,51 @@
|
||||
#---
|
||||
|
||||
function rse_sum(x)
|
||||
s = 0
|
||||
for k = eachindex(x)
|
||||
s = s+x[k]
|
||||
end
|
||||
return s
|
||||
end
|
||||
|
||||
rse_sum(1:36) == 666
|
||||
#---
|
||||
|
||||
function rse_mean(x)
|
||||
return rse_sum(x) / length(x)
|
||||
end
|
||||
|
||||
rse_mean(-15:17) == 1
|
||||
|
||||
#----
|
||||
function rse_std(x)
|
||||
return sqrt(rse_sum((x.-rse_mean(x)).^2)/(length(x)-1))
|
||||
end
|
||||
rse_std([1,2,3]) == 1
|
||||
|
||||
#----
|
||||
function rse_tstat(x;σ = rse_std(x))
|
||||
return rse_mean(x)./ (σ / sqrt(length(x)))
|
||||
end
|
||||
|
||||
rse_tstat(2:3) == 5
|
||||
|
||||
#---
|
||||
struct StatResult
|
||||
x::Vector
|
||||
n::Int32
|
||||
std::Float64
|
||||
tvalue::Float64
|
||||
end
|
||||
length(s::StatResult) = s.n
|
||||
StatResult(x) = StatResult(x,length(x))
|
||||
|
||||
StatResult(x,n) = StatResult(x,n,rse_std(x))
|
||||
StatResult(x,n,std) = StatResult(x,n,)
|
||||
|
||||
|
||||
mystatresult(10,500.) # <1>
|
||||
|
||||
function tstat(x) # <2> generate a function returning our new type
|
||||
return mystatresult(length(x),rse_tstat(x))
|
||||
end
|
@ -1,78 +0,0 @@
|
||||
---
|
||||
type: slide
|
||||
slideOptions:
|
||||
transition: slide
|
||||
width: 1400
|
||||
height: 900
|
||||
margin: 0.1
|
||||
---
|
||||
|
||||
<style>
|
||||
.reveal strong {
|
||||
font-weight: bold;
|
||||
color: orange;
|
||||
}
|
||||
.reveal p {
|
||||
text-align: left;
|
||||
}
|
||||
.reveal section h1 {
|
||||
color: orange;
|
||||
}
|
||||
.reveal section h2 {
|
||||
color: orange;
|
||||
}
|
||||
.reveal code {
|
||||
font-family: 'Ubuntu Mono';
|
||||
color: orange;
|
||||
}
|
||||
.reveal section img {
|
||||
background:none;
|
||||
border:none;
|
||||
box-shadow:none;
|
||||
}
|
||||
</style>
|
||||
|
||||
# Introduction to Research Software Engineering
|
||||
|
||||
---
|
||||
|
||||
## Starting Points
|
||||
|
||||
- [DORA declaration](https://sfdora.org/) in 2012: reshape how research impact should be assessed underlining importance of software
|
||||
|
||||
> For the purposes of research assessment, consider the value and impact of all research outputs (including datasets and software) in addition to research publications ...
|
||||
|
||||
- [UK survey in 2014](https://zenodo.org/record/1183562): 7 out of 10 researchers could not conduct research without software.
|
||||
- [DFG funding calls on research software sustainability](https://www.dfg.de/en/research_funding/programmes/infrastructure/lis/funding_opportunities/call_proposal_software/) in 2016 & 2019 & 2023
|
||||
- [Nationale Forschungsdaten Infrastruktur, NFDI](https://www.nfdi.de/?lang=en) since 2020
|
||||
- Lack of careers for software developers in academia
|
||||
- Lack of reproducibility of research that uses software (*"works for me on my machine"* vs. *"works for everyone everywhere"*)
|
||||
|
||||
---
|
||||
|
||||
## RSE Movement
|
||||
|
||||
... academic software developers needed a name:
|
||||
**Research Software Engineers**
|
||||
|
||||
[UK Society of RSE](https://society-rse.org/):
|
||||
|
||||
> A Research Software Engineer (RSE) combines professional software engineering expertise with an intimate understanding of research.
|
||||
|
||||
- *"Movement"* started in the UK, first UK RSE conference in 2016
|
||||
- First conferences in Germany and the Netherlands in 2019
|
||||
- [de-RSE position paper](https://f1000research.com/articles/9-295/v2) in 2020
|
||||
- Second Thursday of October is the [International RSE Day](https://researchsoftware.org/council/intl-rse-day.html)
|
||||
- [Why be an RSE?](https://researchit.blogs.bristol.ac.uk/2021/10/14/international-rse-day-why-be-an-rse/) Interesting and novel projects, technical freedom, RSEs come from varied backgrounds, development for social good
|
||||
|
||||
---
|
||||
|
||||
## Do I need this in Industry?
|
||||
|
||||
- Yes. Research also happens in industry.
|
||||
- All RSE things we learn (Git, packaging, CI/CD, virtualization, documentation, ...) is also highly relevant for non-research software.
|
||||
- Companies use (more and more) the same workflows and tools.
|
||||
- It is not just about coding. It is about collaborative work.
|
||||
- Open-source development excellent door opener for industry.
|
||||
- Some companies use open-source software and need to make contributions.
|
||||
- Some companies develop their software as open-source software.
|
101
material/1_mon/rse/rse_basics_slides.qmd
Normal file
101
material/1_mon/rse/rse_basics_slides.qmd
Normal file
@ -0,0 +1,101 @@
|
||||
---
|
||||
format: revealjs
|
||||
---
|
||||
|
||||
# Introduction to the Research Software Engineering Summerschol
|
||||
|
||||
## Most important link
|
||||
|
||||
[www.simtech-summerschool.de](https://www.simtech-summerschool.de)
|
||||
|
||||
Find all slides, all materials, and the schedule
|
||||
|
||||
## Last minute organization issues
|
||||
|
||||
-
|
||||
|
||||
-
|
||||
|
||||
## Structure of the summer school
|
||||
|
||||
- Check out the schedule
|
||||
|
||||
- You will learn to use basic Julia
|
||||
|
||||
- In the beginning we will focus on the Research Software Engineering part!
|
||||
|
||||
- Advanced Julia, later this week and by request ;)
|
||||
|
||||
## Your teaching staff I
|
||||
|
||||
|
||||
|
||||
::: {layout-ncol=3 }
|
||||
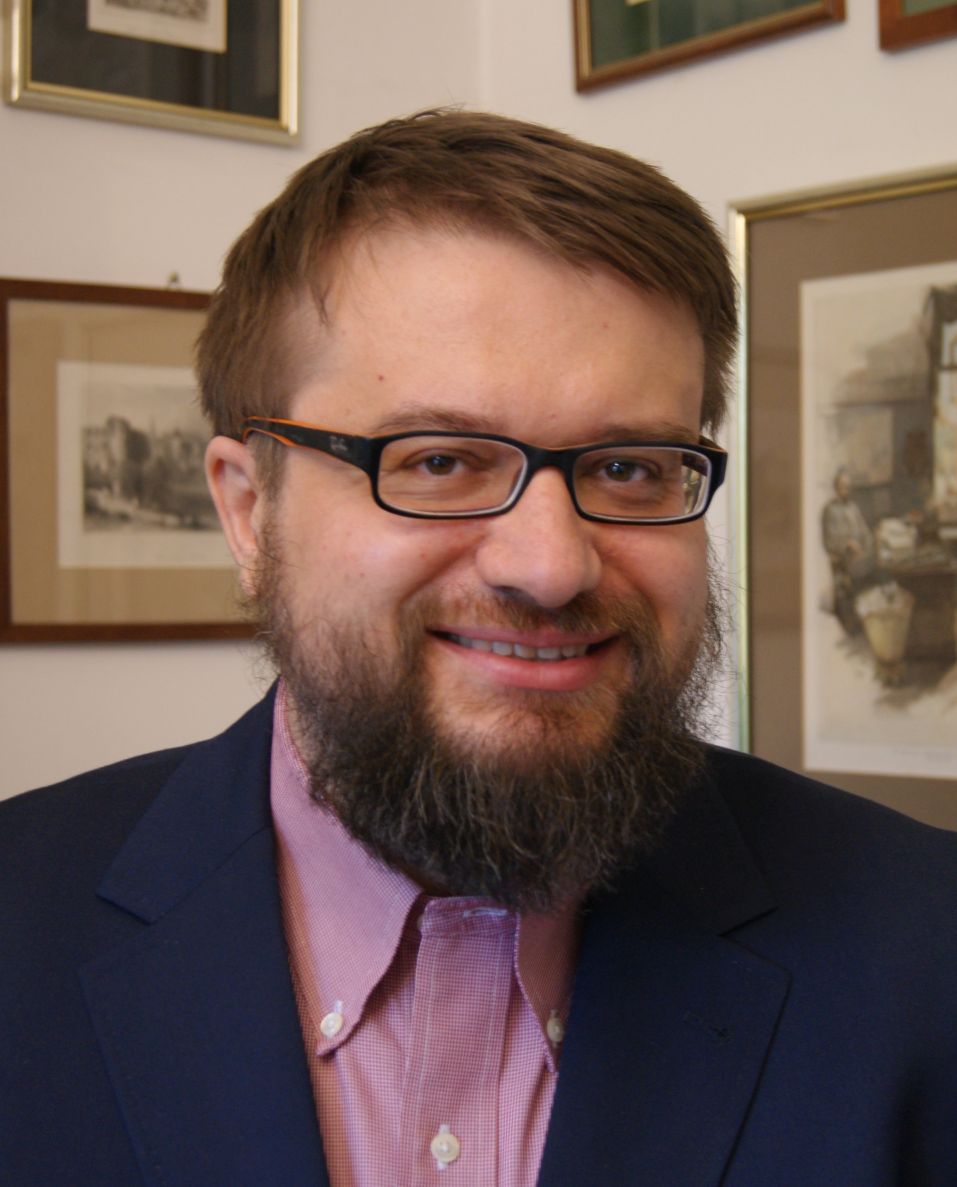
|
||||
|
||||
|
||||
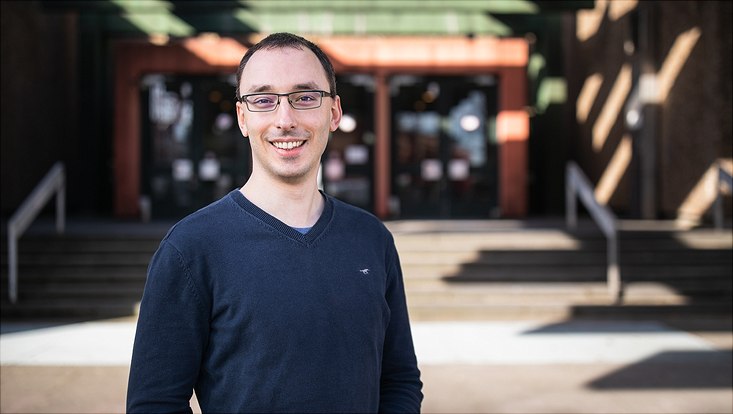
|
||||
|
||||
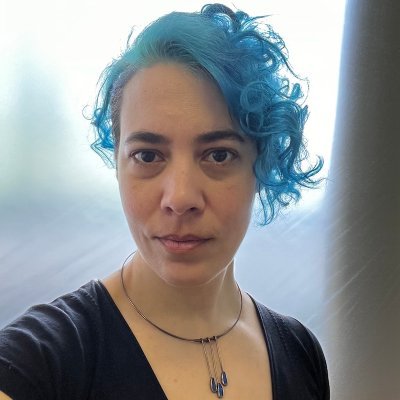
|
||||
:::
|
||||
|
||||
## Your teaching Staff II
|
||||
::: {layout-ncol=3 }
|
||||
|
||||
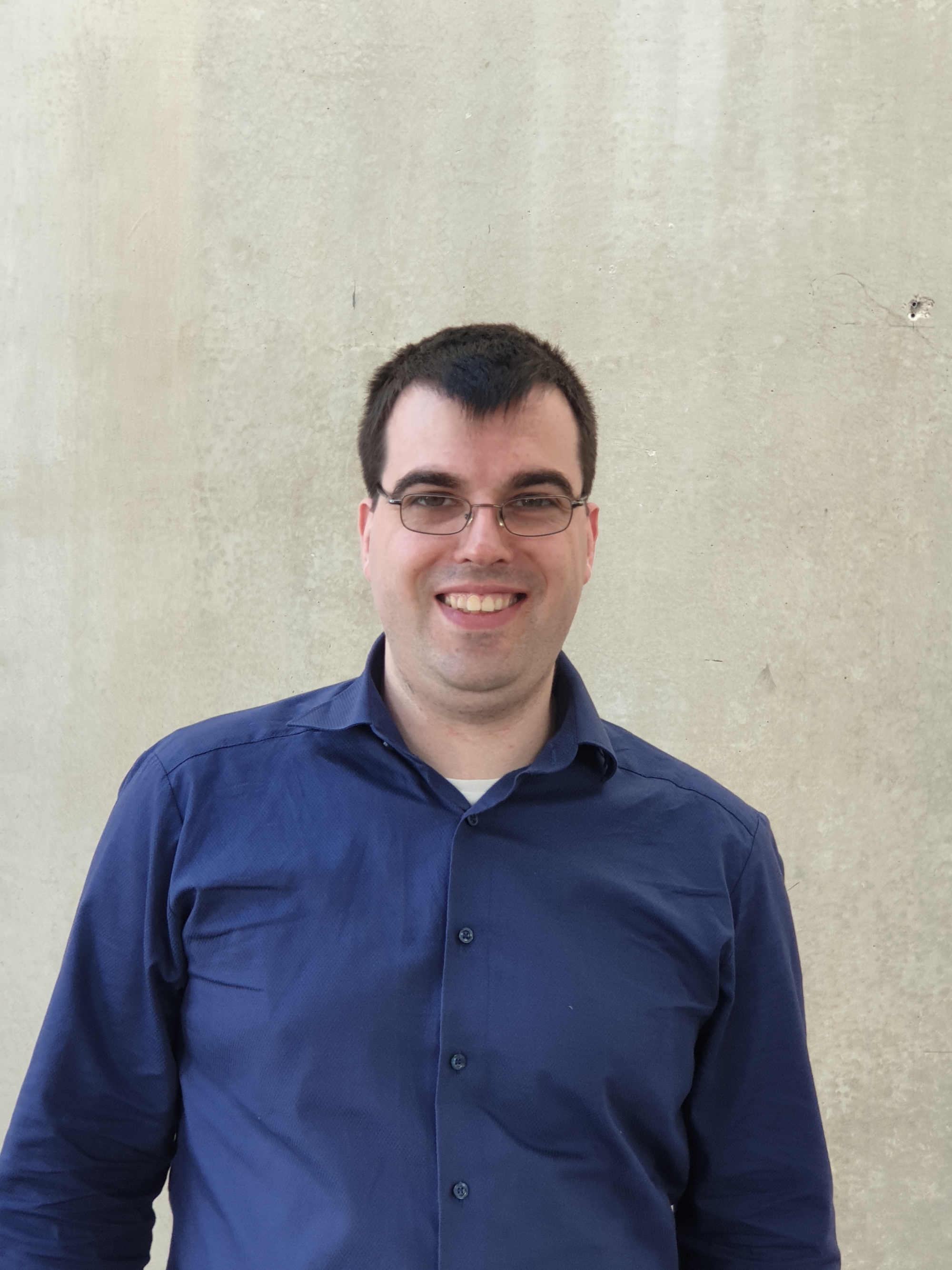
|
||||
|
||||
|
||||
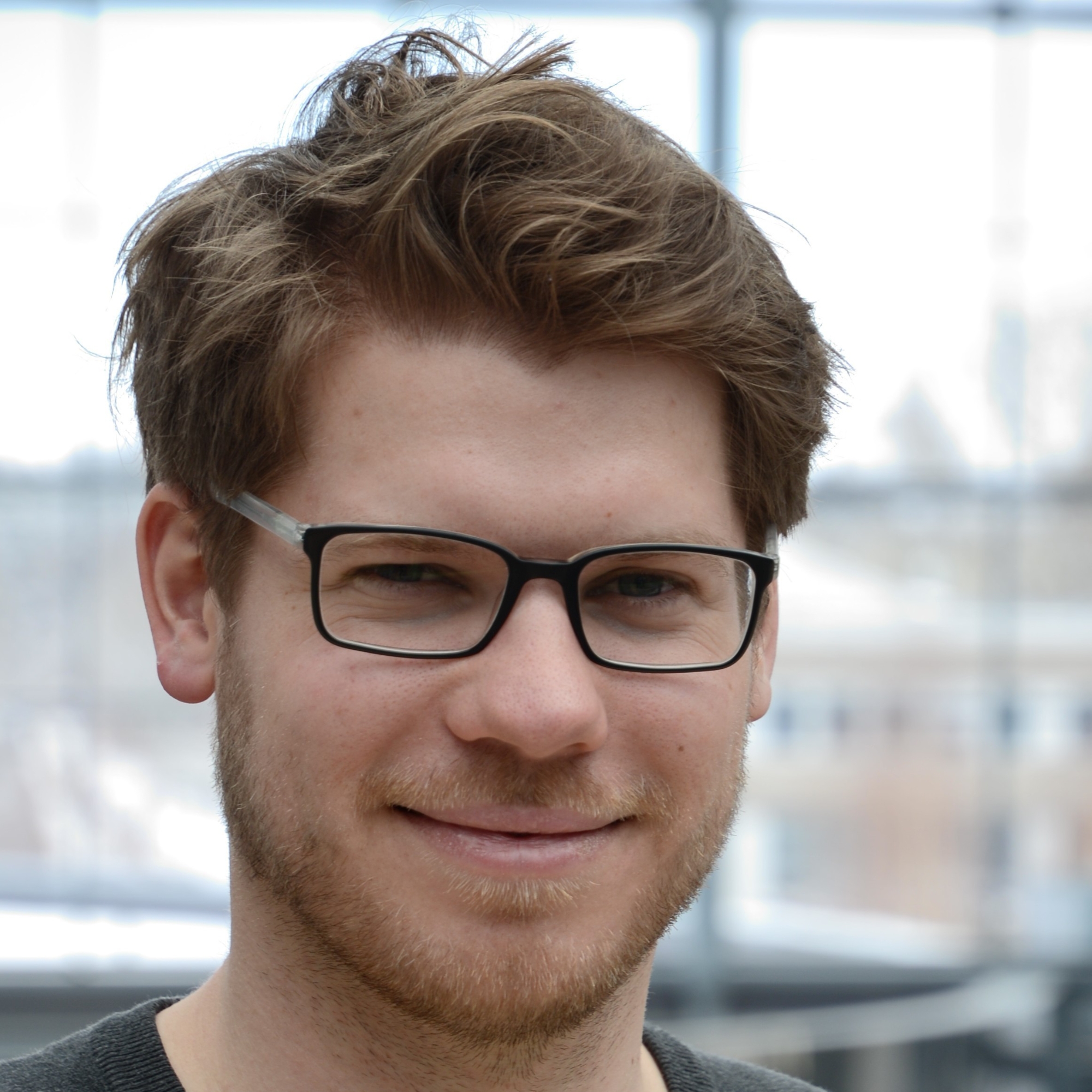
|
||||
|
||||
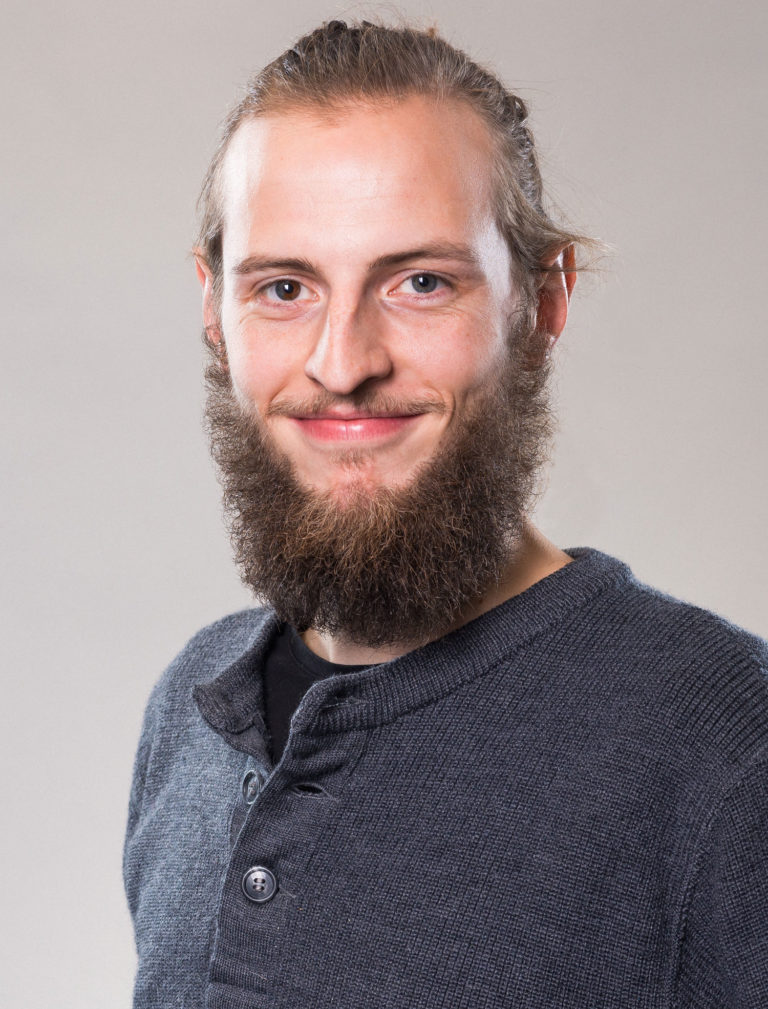
|
||||
|
||||
:::
|
||||
|
||||
|
||||
<!-- ## Your social team -->
|
||||
|
||||
<!-- ## Your admin team -->
|
||||
|
||||
# Introduction to Research Software Engineering
|
||||
|
||||
------------------------------------------------------------------------
|
||||
|
||||
## Starting Points
|
||||
|
||||
- [DORA declaration](https://sfdora.org/) in 2012: reshape how research impact should be assessed underlining importance of software
|
||||
|
||||
> For the purposes of research assessment, consider the value and impact of all research outputs (including datasets and software) in addition to research publications ...
|
||||
|
||||
- [UK survey in 2014](https://zenodo.org/record/1183562): 7 out of 10 researchers could not conduct research without software.
|
||||
- [DFG funding calls on research software sustainability](https://www.dfg.de/en/research_funding/programmes/infrastructure/lis/funding_opportunities/call_proposal_software/) in 2016 & 2019 & 2023
|
||||
- [Nationale Forschungsdaten Infrastruktur, NFDI](https://www.nfdi.de/?lang=en) since 2020
|
||||
- Lack of careers for software developers in academia
|
||||
- Lack of reproducibility of research that uses software (*"works for me on my machine"* vs. *"works for everyone everywhere"*)
|
||||
|
||||
------------------------------------------------------------------------
|
||||
|
||||
## RSE Movement
|
||||
|
||||
... academic software developers needed a name: **Research Software Engineers**
|
||||
|
||||
[UK Society of RSE](https://society-rse.org/):
|
||||
|
||||
> A Research Software Engineer (RSE) combines professional software engineering expertise with an intimate understanding of research.
|
||||
|
||||
- *"Movement"* started in the UK, first UK RSE conference in 2016
|
||||
- First conferences in Germany and the Netherlands in 2019
|
||||
- [de-RSE position paper](https://f1000research.com/articles/9-295/v2) in 2020
|
||||
- Second Thursday of October is the [International RSE Day](https://researchsoftware.org/council/intl-rse-day.html)
|
||||
- [Why be an RSE?](https://researchit.blogs.bristol.ac.uk/2021/10/14/international-rse-day-why-be-an-rse/) Interesting and novel projects, technical freedom, RSEs come from varied backgrounds, development for social good
|
||||
|
||||
------------------------------------------------------------------------
|
||||
|
||||
## Do I need this in Industry?
|
||||
|
||||
- Yes. Research also happens in industry.
|
||||
- All RSE things we learn (Git, packaging, CI/CD, virtualization, documentation, ...) is also highly relevant for non-research software.
|
||||
- Companies use (more and more) the same workflows and tools.
|
||||
- It is not just about coding. It is about collaborative work.
|
||||
- Open-source development excellent door opener for industry.
|
||||
- Some companies use open-source software and need to make contributions.
|
||||
- Some companies develop their software as open-source software.
|
1
missing.md
Normal file
1
missing.md
Normal file
@ -0,0 +1 @@
|
||||
This content is not yet included in the website - check at another time!
|
34
schedule.qmd
34
schedule.qmd
@ -6,26 +6,22 @@ title: "Schedule"
|
||||
The schedule is very preliminary and will most likely change drastically :)
|
||||
:::
|
||||
|
||||
| | Sunday | Monday | Tuesday | Wednesday | Thursday | Friday |
|
||||
| | Sunday | Monday | Tuesday | Wednesday | Thursday | Friday |
|
||||
|-----------|-----------|-----------|-----------|-----------|-----------|-----------|
|
||||
| | Arrival | Basics of RSE and Julia | Julia+RSE | Julia+Viz+Simulation | Stats + Projects | Optimization + Project |
|
||||
| | | | | | | |
|
||||
| 09.00 | | | **Benjamin**: Advanced Git / Contributing (1+1) | **Hendrik**: All the nice Julia Features (1+1)? | **Marco** Resampling: Bootstrap & Permutation tests (2+2) | **Doug**: Optimized (bootstrap) code (1)? |
|
||||
| 10.00 | | **Benjamin**: Welcome / What and why RSE? (1) | **Benjamin/Bene**: Unittests (1+1) | **Bene** Vizualization in Julia (1+1) | ~~~ | Project Work |
|
||||
| 11.00 | | **Hendrik**: Why Julia? (1)? | ~~~~ | ~~~ | | cont. |
|
||||
| 12.00 | | **Bene**: First Steps - "Hello World" + Types (1+1) | ~~~~ | ~~~ | | |
|
||||
| 13.00 | | **Lunch** | **Lunch** | **Lunch** | **Lunch** | **Lunch** |
|
||||
| 14.00 | | ~~~ |**Benjamin**: Continuous Integration (1+1)| **Lisa**: Simulating Data (1+1) | Project Work | Project Work |
|
||||
| 15.00 | | **Bene**: Julia + Git + Packages/Environments (1+1) | **Lisa**: Code-Review (1+1)? | ~~~ | | cont. |
|
||||
| 16.00 | |~~~ | ~~~ | **Doug**: Things to keep in mind for datastorage (1+1)? | | |
|
||||
| 17.00 | | **Bene**: MyFirstPackage.jl (1+1) | ~~~ | ~~~ | | Farewell |
|
||||
| 18.00 | | ~~~ | **Bene**: PkgTemplates.jl (0.5+0.5) => wed/hendrik? | ~~~ | | |
|
||||
| 19.00 | Pre-Get-together | Welcome-BBQ \@ SimTech | Social 1 | Social 2 | Social 3 | |
|
||||
|
||||
|
||||
| | Arrival | Basics of RSE and Julia | Julia+RSE | Julia+Viz+Simulation | Stats + Projects | Optimization + Project |
|
||||
| | | | | | | |
|
||||
| 09.00 | | | **Benjamin**: Advanced Git / Contributing (1+1) | **Hendrik**: All the nice Julia Features (1+1)? | **Marco** Resampling: Bootstrap & Permutation tests (2+2) | **Doug**: Optimized (bootstrap) code (1)? |
|
||||
| 10.00 | | **Benjamin**: Welcome / What and why RSE? (1) | **Benjamin/Bene**: Unittests (1+1) | **Bene** Vizualization in Julia (1+1) | \~\~\~ | Project Work |
|
||||
| 11.00 | | **Hendrik**: Why Julia? (1)? | \~\~\~\~ | \~\~\~ | | cont. |
|
||||
| 12.00 | | **Bene**: First Steps - "Hello World" + Types (1+1) | \~\~\~\~ | \~\~\~ | | |
|
||||
| 13.00 | | **Lunch** | **Lunch** | **Lunch** | **Lunch** | **Lunch** |
|
||||
| 14.00 | | \~\~\~ | **Benjamin**: Continuous Integration (1+1) | **Lisa**: Simulating Data (1+1) | Project Work | Project Work |
|
||||
| 15.00 | | **Bene**: Julia + Git + Packages/Environments (1+1) | **Lisa**: Code-Review (1+1)? | \~\~\~ | | cont. |
|
||||
| 16.00 | | \~\~\~ | \~\~\~ | **Doug**: Things to keep in mind for datastorage (1+1)? | | |
|
||||
| 17.00 | | **Bene**: MyFirstPackage.jl (1+1) | \~\~\~ | \~\~\~ | | Farewell |
|
||||
| 18.00 | | \~\~\~ | **Bene**: PkgTemplates.jl (0.5+0.5) =\> wed/hendrik? | \~\~\~ | | |
|
||||
| 19.00 | Pre-Get-together | Welcome-BBQ \@ SimTech | Social 1 | Social 2 | Social 3 | |
|
||||
|
||||
: {.striped .hover}
|
||||
|
||||
|
||||
Missing Topics right now: Documentation, License, MultipleRegression?
|
||||
Simulating Data - Requirements regarding regression?
|
||||
Missing Topics right now: Documentation, License, MultipleRegression? Simulating Data - Requirements regarding regression?
|
10
teaching.qmd
10
teaching.qmd
@ -4,17 +4,17 @@ title: "Teachers"
|
||||
|
||||
## Invited Speakers
|
||||
|
||||
[Prof. Lisa DeBruine](https://debruine.github.io/) - Julia, Statistics, Simulation
|
||||
[Prof. Lisa DeBruine](https://debruine.github.io/) - Statistics, Simulation
|
||||
|
||||
[Prof. Doug Bates](https://github.com/dmbates) - Julia, Statistics, Optimization
|
||||
[Prof. Przemysław Szufel]() - Julia, Optimization
|
||||
|
||||
[Prof. Hendrik Ranocha](https://ranocha.de) - Julia, RSE, Optimization
|
||||
|
||||
|
||||
## Organizers and Teachers
|
||||
|
||||
[Marco Oesting](https://www.isa.uni-stuttgart.de/computational-statistics/) - Statistics
|
||||
[Assistant Prof. Marco Oesting](https://www.isa.uni-stuttgart.de/computational-statistics/) - Statistics
|
||||
|
||||
[Benjamin Uekermann](https://github.com/uekerman) - RSE
|
||||
[Assistant Prof. Benjamin Uekermann](https://github.com/uekerman) - RSE
|
||||
|
||||
[Benedikt Ehinger](https://www.s-ccs.de/) - Julia, Visualization, RSE
|
||||
[Assistant Prof. Benedikt Ehinger](https://www.s-ccs.de/) - Julia, Visualization, RSE
|
||||
|
Loading…
x
Reference in New Issue
Block a user